Based on customer requests we've examined ways to update Portable Apps, like apps form the SysInternals bundle.
There are many ways to achieve this, but we wanted one that works well with the Custom Software feature in VulnDetect.
We also wanted to avoid making one Config per App in the SysInternals bundle.
And, since these files can live in various places, including folders that are writable by non-privileged users, we wanted to be careful not to overwrite the wrong files or follow symbolic links.
The script will not overwrite files unless they have the company name specified in the $companyNameToCheck
variable, it will also not touch files in the $excludedDirs
.
The combination of Custom Software and this script assumes that you add a ZIP archive as an Additonal File and that the ZIP archive contains a folder called "files" with all the SysInternal files you want to replace, e.g. the entire SysInternals bundle.
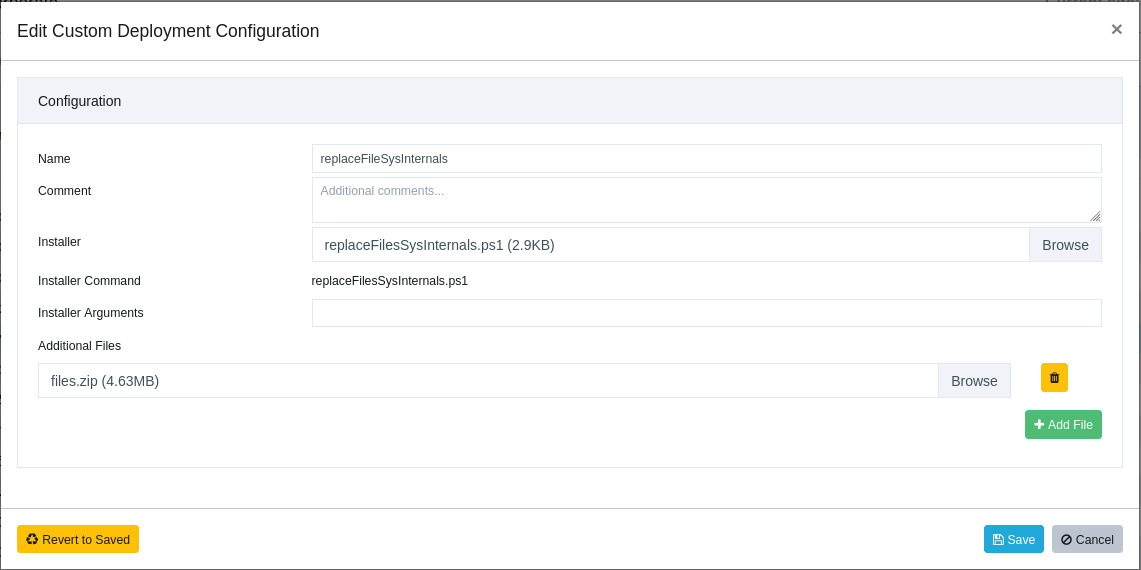
You can also edit the $sourceDir
variable if you want to use a different layout in your ZIP archive.
The below script was created by ChatGPT (by OpenAI). It was created based on the above requirements and it has been reviewed and tested by SecTeer.
Note: This script is intended to run in an automated fashion and with a sub folder of known trusted files. Executing this in the wrong location and altering variables and conditions may have unforeseen consequences, because the script overwrites files recursively.
# Define the company name to check for
$companyNameToCheck = "Sysinternals - www.sysinternals.com"
# Get the current working directory and set the source directory to the "files" subfolder
$sourceDir = Join-Path -Path (Get-Location) -ChildPath "files"
$drive = "C:\"
# List of directories to exclude (common shim file locations)
$excludedDirs = @(
"$env:SystemRoot\AppPatch",
"$env:SystemRoot\System32\ShimCache"
)
# Function to get the latest file from a directory
function Get-LatestFile {
param (
[string]$directory,
[string]$fileName
)
$files = Get-ChildItem -Path $directory -Filter $fileName
$latestFile = $files | Sort-Object LastWriteTime -Descending | Select-Object -First 1
return $latestFile
}
# Function to check the "Company Name" and "Product Name" properties of a file
function Get-FileProperties {
param (
[string]$filePath
)
$properties = Get-ItemProperty -Path $filePath -Name 'VersionInfo'
return @{
CompanyName = $properties.VersionInfo.CompanyName
ProductName = $properties.VersionInfo.ProductName
}
}
# Function to recursively replace files in the target directory with the latest from the source directory
function Replace-Files {
param (
[string]$sourceDir,
[string]$drive,
[string]$companyNameToCheck
)
# Get the list of files in the source directory
$sourceFiles = Get-ChildItem -Path $sourceDir
# Iterate over each file in the source directory
foreach ($sourceFile in $sourceFiles) {
# Get the latest version of the source file
$latestSourceFile = Get-LatestFile -directory $sourceDir -fileName $sourceFile.Name
# Find matching files in the drive recursively, excluding the source directory and excluded directories
$matchingFiles = Get-ChildItem -Path $drive -Filter $sourceFile.Name -Recurse -ErrorAction SilentlyContinue | Where-Object {
$_.FullName -notlike "$sourceDir*" -and
$excludedDirs -notcontains $_.DirectoryName
}
# Replace each matching file with the latest source file if the company name matches and it is not a Chocolatey shim file
foreach ($targetFile in $matchingFiles) {
$properties = Get-FileProperties -filePath $targetFile.FullName
if ($properties.CompanyName -eq $companyNameToCheck -and $properties.ProductName -notlike "*Chocolatey Shim*") {
Copy-Item -Path $latestSourceFile.FullName -Destination $targetFile.FullName -Force
Write-Output "Replaced $($targetFile.FullName) with $($latestSourceFile.FullName)"
} else {
Write-Output "Skipped $($targetFile.FullName) as it is either not from '$companyNameToCheck' or it is a Chocolatey shim file"
}
}
}
}
# Call the function to replace files
Replace-Files -sourceDir $sourceDir -drive $drive -companyNameToCheck $companyNameToCheck